Competitive Programming Course
Live Instructor-Led Classes with Project-Based Learning in Competitive Programming Course
- 6-Month Training
- 2-Year LMS Access
- A Dedicated Job Application Portal



Course Highlights
Weekly Live Sessions
Doubt Clarification Sessions
Hands-on Coding Exercises
A Dedicated Success Coach
Master classes by Industry Experts
2-Year LMS access
Certificate of Accomp-lishment
Flexible EMI Options
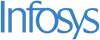
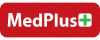
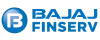
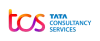
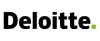
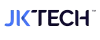
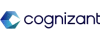


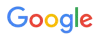

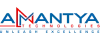
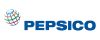
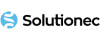
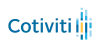
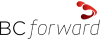
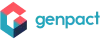

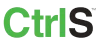


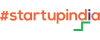
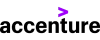
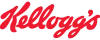
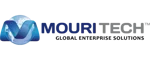
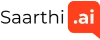
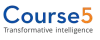

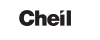
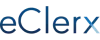

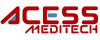
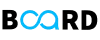
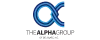
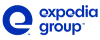


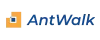
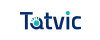
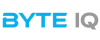
Competitive Programming Course Overview
Do you want to sharpen your problem-solving skills and excel in coding competitions or technical interviews at top tech companies?
Our Competitive Programming Course is a live, instructor-led program designed to turn you into a coding expert in just 6 months. This course focuses on enhancing your algorithmic thinking and problem-solving abilities, preparing you for competitive programming challenges and coding interviews. You’ll learn to tackle a wide range of problems using data structures (arrays, trees, graphs) and advanced algorithms (dynamic programming, greedy algorithms, recursion), while optimizing your code for performance and efficiency.
With extensive hands-on coding practice on popular platforms like Codeforces, LeetCode, and HackerRank, you’ll build the speed and accuracy needed to succeed in contests and solve complex problems under time constraints. You’ll also learn strategies for improving time and space complexity, helping you write optimized, scalable code for real-world scenarios.
Reach out to a career counselor today to check your eligibility and get started on the path to becoming a top competitive programmer!
Career Services in a nutshell
Get ready for your dream job! Attend comprehensive industry readiness training with Career Services.
- Communication and aptitude training
- Mock interviews
- 200+ jobs available on our placement portal
- Workshops on workplace behaviour
Learn workplace skills!
Your working style and behavior decide if you are a good cultural fit. Learn the right skills so you never feel out of place at work.
Sell your skills, and sell them well.
You are halfway there if you have a great resume and an optimized online presence. Work with our experts to build your professional profiles.
Attend mock interviews!
Get a grip on those pre-interview nerves. Test and practice your skills with mock interview sessions.
Competitive Programming Course Syllabus
An industry-aligned curriculum to make you productive from day one – we update the curriculum every month to make sure you learn the most in-demand skills.
- Introduction to the Course
- What is Competitive Programming
- Competitive Programming Competitions
- Websites for Competitive Programming
- Terminologies in Competitive Programming
- Choosing a Programming Language
- Taking Input & Output in Competitive Programming Contests
- Cout vs Printf
- Endl vs Back n
- Fast Cin Cout Methods _ Conclusion
- Time Complexity in Competitive Programming
- Calculating Time Complexity of Code
- Importance of English in Competitive Programming
- Introduction to STL in C++
- Standard Array
- Standard Vector
- Standard Set
- Standard Multiset
- Standard Map
- Standard Multimap
- Standard Pair
- Standard Forward List
- Standard List
- Standard Unordered Set
- Standard Unordered Multiset
- Unordered Map and Unordered Multimap
- Standard Stack
- Standard Queue
- Standard Priority Queue
- Standard Deque
- Importance of Bit Manipulation Techniques
- Basic Binary Operations
- Bit Operations in C++
- Check ith bit is SET or NOT SET
- Toggle ith bit
- Check odd or even using bits
- Check if Number is Power of 2
- Need for Modulo 10^9 + 7
- Modulo Arithmetic
- [A3.1] Problem XOR ORED
- [A3.2] Solution – XOR-ORED
- [A3.3] Solution with Code – XOR-ORED
- Introduction to Graphs
- Weights in Graphs
- Why Study Graphs
- Edge List
- Adjacenty Matrix
- Adjacenty List
- DFS Traversal
- Implementing DFS Traversal
- BFS Traversal
- Implementing BFS Traversal
- Minimum Spanning Tree
- Concept of Kruskal_s Algorithm
- Concept of Prim_s Algorithm
- Concept of Dijkstra Algorithm
- [A4.1] Problem – CHFPLN
- [A4.2] Solution – CHFPLN
- [A4.3] Solution with Code – CHFPLN
- Sum of Updated Range Problem
- Solving Sum of Range Problem with Segment Trees
- Segment Trees – Update Queries
- Segment Trees – Calculate Sum
- Implementing Segment Tree – Part 1
- Implementing Segment Tree – Part 2
- Problem – Range Minimum Query
- Implementing Range Minimum Query
- Implementing Segment Tree – Tricks
- Lazy Propagation in Segment Trees – Part 1
- Lazy Propagation in Segment Trees – Part 2
- Implementing Lazy Propagation in Segment Trees – Part 1
- Implementing Lazy Propagation in Segment Trees – Part 2
- Problem – Xenia and Bit Operations
- Solution – Xenia and Bit Operations
- Solution with Code – Xenia and Bit Operations
- Problem – Circular RMQ
- Solution with Code – Circular RMQ – Part 1
- Solution with Code – Circular RMQ – Part 2
- Introduction to Backtracking
- N Queens Problem Explained
- N Queens Solved
- Knights Tour Problem Explained
- Knight’s Tour Solved
- Rat in Maze Problem Explained
- Rat in Maze Solved
- Problem Richie Rich
- Solving Richie Rich
- Problem False Number
- Solving False Number
- Algorithms
- Introduction to Greedy Algorithms
- Understanding Greedy with Fractional Knapsack Problem
- Solving Fractional Knapsack Problem
- Tasks and Deadlines Problem
- Solving Tasks and Deadlines Problem
- Optimal File Merging Problem
- Solving Optimal File Merge Patterns Problem
- Huffman Coding
- [A7.1] Problem – Dragons
- [A7.2] Solution with Code – Dragons
- [A7.3] Problem – Little Elephant and Bits
- [A7.4] Solution with Code – Little Elephant and Bits
- Introduction to Dynamic Programming
- Top down vs Bottom Up
- Fibbonacci Problem Top Down
- Implementing Fibonnaci with DP
- 2D Grid Traversal Problem
- Implementing 2D Grid Traversal Problem
- Understanding Memoization
- Fibbonacci with Tabulation
- Implementing Fibbonacci with Tabulation
- 2D Grid Traversal Problem with Tabulation
- Implementing 2D Grid Traversal Problem with Tabulation
- Understanding Tabulation
- [A8.1] Problem – Subset Sum Problem
- [A8.2] Solving – Subset Sum Problem
- Binary Lifting (Kth Ancestor of a Tree Node)
- LCA (Lowest Common Ancestor) Problem
- Fenwick Trees – Introduction
- Fenwick Trees – Sum of Range (Range Find Query)
- Fenwick Trees – Update (Point Update Query)
- Problem – Distance between nodes in Trees
- Solution – Distance between nodes in Trees
- Implementing Fenwick Trees
- Implementing Binary Uplifting
- Implementing LCA
- Solution with Code – Distance between nodes in Trees
Tools and Technologies
Let us help you become an industry asset
Attend job readiness training along with your technical training.
Master Competitive Programming with
- Live Online Classes
- Doubt Clarification
- Profile Building
- Career Services
Step 1
Apply
Step 2
Talk to A Counsellor
Step 3
Review Your Eligiblity
Step 4
Get Started
Competitive Programming Course Fee
Option 1: One Time Payment
- Early Bird Offer Up To ₹10,000
- Offer only valid for the first 100 seats!
Option 2: Pay In EMI
Discuss with Your Mentors
Showcase your certificate as a symbol of your web development expertise.
