Java Spring Boot Course
Live Instructor-Led Classes with Project-Based Learning in Java Spring Boot Course
- 6-Month Training
- 2-Year LMS Access
- A Dedicated Job Application Portal




Course Highlights
Weekly Live Sessions
Doubt Clarification Sessions
Hands-on Coding Exercises
A Dedicated Success Coach
Master classes by Industry Experts
2-Year LMS access
Certificate of Accomp-lishment
Flexible EMI Options
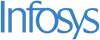
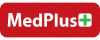
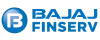
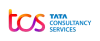
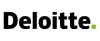
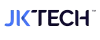
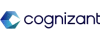


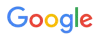

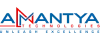
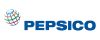
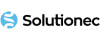
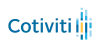
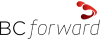
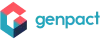

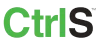


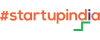
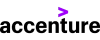
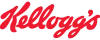
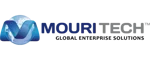
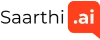
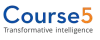

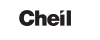
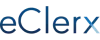

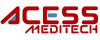
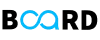
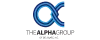
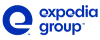


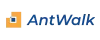
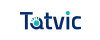
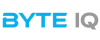
Java Spring Boot Course Overview
Are you ready to master the Java Spring Boot framework and build robust, enterprise-grade applications?
Our Java Spring Boot Developer Course is a live, instructor-led program designed to equip you with the skills to build powerful back-end applications in just 6 months. You’ll learn the fundamentals of Java and dive deep into the Spring Boot framework, which is widely used for creating scalable, production-ready applications. The course covers essential concepts such as dependency injection, RESTful APIs, database integration with Hibernate, and Spring Data JPA.
Contact a career counselor today to check your eligibility and start your journey to becoming a proficient Java Spring Boot developer!
Career Services in a nutshell
Get ready for your dream job! Attend comprehensive industry readiness training with Career Services.
- Communication and aptitude training
- Mock interviews
- 200+ jobs available on our placement portal
- Workshops on workplace behaviour
Learn workplace skills!
Your working style and behavior decide if you are a good cultural fit. Learn the right skills so you never feel out of place at work.
Sell your skills, and sell them well.
You are halfway there if you have a great resume and an optimized online presence. Work with our experts to build your professional profiles.
Attend mock interviews!
Get a grip on those pre-interview nerves. Test and practice your skills with mock interview sessions.
Java Spring Boot Course Syllabus
An industry-aligned curriculum to make you productive from day one – we update the curriculum every month to make sure you learn the most in-demand skills.
- Course Introduction
- [Important] Access for Source Code and Learner Community
- Introduction to Java
- JDK Setup
- First Code in Java
- How Java Works
- Variables
- Data Types
- Literal
- Type Conversion
- Arithmetic Operators
- Relational Operators
- Logical Operators
- If Else
- If Else If
- Ternary
- Switch Statement
- Need For Loop
- While Loop
- Do While Loop
- For Loop
- Which Loop To Use
- Class And Object Theory
- Class and Object Practical
- JDK JRE JVM
- Methods
- Method Overloading
- Stack And Heap
- Need of Array
- Creation of Array
- Multi Dimensional Array
- jagged and 3D Array
- Drawbacks of Array
- Array of Objects
- Enhanced for loop
- What is String
- Mutable vs Immutable string
- StringBuffer and StringBuilder
- Static Variable
- Static method
- Static block
- Encapsulation
- Getters and setters
- This keyword
- Constructor
- Default vs Parameterized Constructor
- Naming Convention
- Anonymous Object
- What is Inheritance
- Need of Inheritance
- Single and Multilevel inheritance
- Multiple Inheritance
- This and super method
- Method Overriding
- Packages
- Access Modifiers
- Polymorphism
- Dynamic Method Dispatch
- Final keyword
- Object Class equals toString hashcode
- Upcasting and Downcasting
- Wrapper Class
- Introduction
- Abstract keyword
- Inner class
- Anonymous Inner class
- Abstract and Anonymous Inner Class
- What is Interface
- More on Interfaces
- Need of Interface
- What is Enum
- Enum if and switch
- Enum Class
- What is Annotation
- Types of Interface
- Functional Interface New
- Lambda Expression
- Lambda Expression with return
- What is Exception ?
- Exception Handling using try catch
- Try with multiple catch
- Exception Hierarchy
- Exception throw keyword
- Custom Exception
- Ducking Exception using throws
- User Input using Buffered Reader and Scanner
- Try with resources
- Threads
- Multiple Threads
- Thread Priority and Sleep
- Runnable vs Thread
- Race Condition
- Thread states
- Collection API
- ArrayList
- Set
- Map
- Comparator vs Comparable
- Need of Stream API
- forEach Method
- Stream API
- Map Filter Reduce Sorted
- ParallelStream in Java
- Optional class in Java
- Method Reference
- Constructor Reference
- Maven introduction
- Maven in IDE
- Getting Dependencies
- Effective POM
- Maven Archetype
- Maven in Eclipse
- How Maven Works
- JDBC Introduction
- Postgres Setup
- JDBC Steps
- Postgres Library jar
- Connecting Java and DB
- Execute and Process
- Fetching all Records
- Crud operations
- Problems with Statement
- Prepared Statement
- Introduction To Spring
- Spring Docs
- Prerequisites
- IDE For Spring
- IoC And DI
- Spring Vs Spring Boot
- First Spring Boot App
- DI Using Spring Boot
- Autowiring In Spring Boot
- Spring 1st Project
- Spring Bean Xml Config
- Object Creation
- Scopes
- Setter Injection
- Ref Attribute
- Constructor Injection
- Creating Interface
- Autowiring
- Primary Bean
- Lazy Init Bean
- Getbean By Type
- Inner Bean
- Java Based Config
- Bean Name
- Scope Annotation
- Autowire
- Primary And Qualifier
- Component Stereotype Annotation
- Autowire Field, Constructor, Setter
- Primary Annotation
- Scope And Value Annotation
- Spring To Spring Boot
- Using Annotations In Spring Boot
- Different Layers
- Service Class
- Repository Layer
- Spring JDBC Introduction
- Creating A Spring JDBC Project
- Student Service And Repo
- JDBC template
- Schema And Data Files
- Rowmapper
- Spring JDBC Postgres
- Web App Introduction
- Creating A Servlet Project
- Running Tomcat
- Servlet Mapping
- Responding To The Client
- Introduction to MVC
- Creating a Spring Boot Web App Project
- Creating a JSP Page
- Creating a Controller
- Request Mapping
- Sending data to Controller
- Accepting Data the servlet way
- Display Data on Result Page
- RequestParam
- Model Object
- Setting Prefix and Suffix
- Model And View
- Need for Model Attribute
- Using Model Attribute
- Spring MVC Introduction
- Creating a Spring MVC Project
- Running Tomcat in Eclipse
- Dispatcher Servlet
- Configuring the Dispatcher Servlet
- Internal Resource View Resolver
- Summary
- Building Job App
- Creating a Project
- Understanding Views
- Home and AddJob Controller
- Handling Form
- Working with Layers
- View Data
- Summary for Job Webapp
- Rest using Spring Boot Introduction
- What is REST
- Http Methods
- Understanding the React UI
- Working with Postman
- Creating a Rest Controller
- Path Variable
- Connecting React and Spring
- Sending Data and RequestBody
- Put and Delete Mapping
- Content Negotiation
- Spring Data JPA Introduction
- What is ORM and JPA
- Creating Table And Inserting Data
- Findall
- findById
- Query DSL
- Update and Delete
- JPA in Job App
- Loading Data and Entity
- Search by Keyword
- React UI for Search
- React UI for Update and Delete
- Project Introduction
- Running and Understanding the React UI Code
- Spring Boot Project Setup
- Creating Product Model and Table
- Fetching All Products from DB
- ResponseEntity
- Fetch Product By Id
- Add Product with Image
- Fetch Product Image
- Update and Delete Product
- Search
- Spring Data Rest Introduction
- Creating A Data Rest Project
- Running The Project
- Update And Delete
- Spring AOP Introduction
- Logging the Calls
- AOP Concepts
- Before Advice
- JoinPoint
- After Advice
- Performance Monitoring using Around Advice
- Validating the input using Around Advice
- Importance Of Security
- OWASP Top 10
- Creating a Spring Security Project
- default login form
- Spring Security Filters
- Session ID
- Setting username and password
- Basic Auth using Postman
- What is CSRF
- Error without CSRF Token
- Sending CSRF Token
- Same Site Strict
- Security Configuration
- Disabling CSRF Token
- without lambda
- Getting ready for user database
- Working with Multiple Users
- Creating User table and db properties
- AuthenticationProvider
- Creating a UserDetailsService
- User Repository
- UserDetails and UserPrincipal
- Summary till now
- What is Bcrypt
- User Registration
- BCrypt Encoding for User Registration
- Setting Password Encoder
- Plan to secure Job App Project
- Cross Origin
- Adding Security Configuration
- Job App is secure now
- Encryption and Decryption
- Project Setup for JWT
- Why JWT
- What is JWT
- Custom Login
- Generating token
- Token Generated
- Creating a JWT Filter
- Setting AuthToken in SecurityContext
- Validating token
- JWT Summary
- Implementing OAuth2
- Google Oauth2 Login
- Github Login
- Docker Introduction
- What Problem We Are Trying To Solve
- Solution With Virtualization
- Solution With Containerization
- What is Docker
- Docker Setup
- Running First Container
- Docker Commands
- Docker Architecture
- Running JDK Docker Container
- Packing The Spring Boot Web App
- Running Spring Boot Web App On Docker
- Docker File For Docker Images
- Web App With Postgres
- Docker Compose
- Running Multiple Containers
- Docker Volumes
- What is Cloud
- Which cloud
- AWS account signup process
- AWS services and IAM account
- Simple Web App Project
- Deploying on Elastic Beanstalk
- Spring Project with DB
- Creating database in AWS RDS
- Deploying app on Beanstalk
- Introduction to ECS
- Configuring AWS CLI
- Creating Cluster and Task
- Running the task for Postgres
- Pushing the docker image to ECR
- Running Java App Task
Tools and Technologies
Let us help you become an industry asset
Attend job readiness training along with your technical training.
Master Java & Spring Boot with
- Live Online Classes
- Doubt Clarification
- Profile Building
- Career Services
Step 1
Apply
Step 2
Talk to A Counsellor
Step 3
Review Your Eligiblity
Step 4
Get Started
Java Spring Boot Developer Course Fee
Option 1: One Time Payment
- Early Bird Offer Up To ₹10,000
- Offer only valid for the first 100 seats!
Option 2: Pay In EMI
Discuss with Your Mentors
Showcase your certificate as a symbol of your web development expertise.
